Wave fields experiment
Links
- Code repository: https://gitlab.com/autotel/wave-fields
- Live version to try out: https://autotel.co/wave-fields/
First attempt with OpenFrameworks
The first version, programmed in Openframeworks worked, but programming good user interfaces is a big challenge. This is why I decided to use Javasript instead.
The public repository for this code is: https://gitlab.com/autotel/envelope-fields-composer
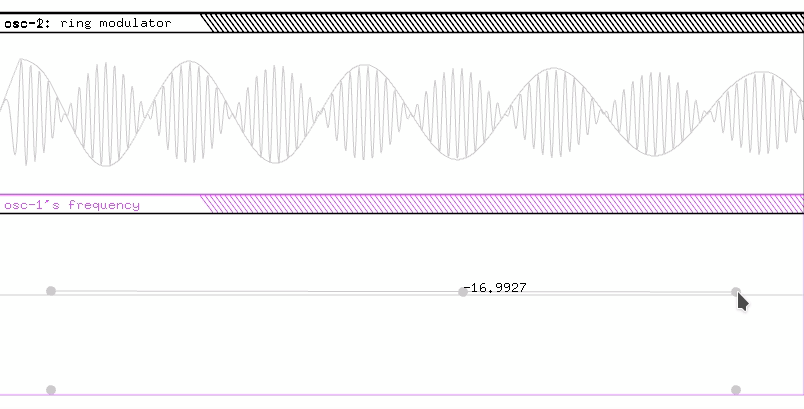
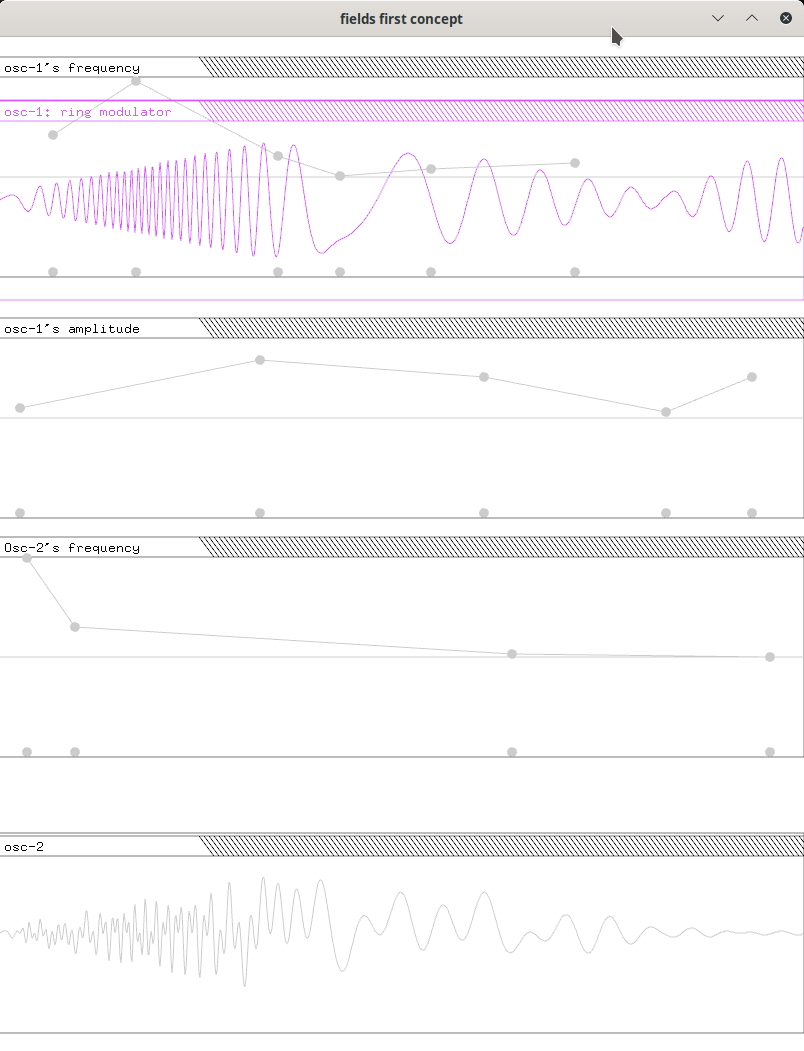
Javascript Version.
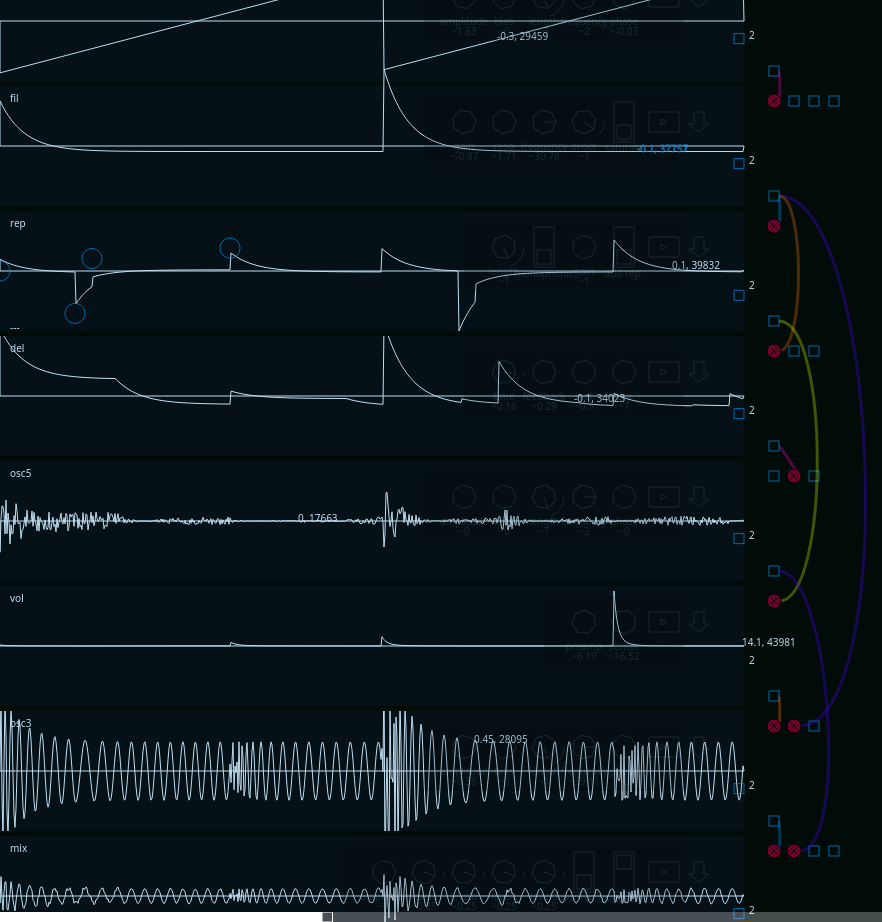
With Javascript, it's also possible to use workers, that can calculate the audio samples much faster. This code features a custom made model abstractions library, that helps creating notifications of changes between different parts of the user interface with the sound processing mechanisms. Another difficult to implement feature was a combination of the modular exchange of waves between the modules or fields in combination with asynchronous calculation of the samples.
Videos
Only for the brave
Hopefully I get time to make a tutorial of how to use this experiment, and more complete documentation of the code. It's not easy nor simple. But in case that you are good figuring out things, here is an example (this goes into the web inspector):
let osc = create(possibleModules.Oscillator,'osc');
let fil = create(possibleModules.Filter,'fil');
let rep = create(possibleModules.Repeater,'rep');
let del = create(possibleModules.Delay,'del');
let osc5 = create(possibleModules.Oscillator,'osc5');
let vol = create(possibleModules.VoltsPerOctaveToHertz,'vol');
let osc3 = create(possibleModules.Oscillator,'osc3');
let mix = create(possibleModules.Mixer,'mix');
osc.outputs.main.connectTo(fil.inputs.main);
fil.outputs.main.connectTo(osc3.inputs.amplitude);
fil.outputs.main.connectTo(del.inputs.main);
fil.outputs.main.connectTo(rep.inputs.main);
rep.outputs.main.connectTo(vol.inputs.main);
del.outputs.main.connectTo(osc5.inputs.amplitude);
osc5.outputs.main.connectTo(mix.inputs.b);
vol.outputs.main.connectTo(osc3.inputs.frequency);
osc3.outputs.main.connectTo(mix.inputs.a);
osc.set({
'amplitude': 1.6300000000000001,
'bias': 0,
'length': 1,
'frequency': 2,
'phase': -0.03,
'shape': 'ramp'
});
fil.set({
'gain': -0.8700000000000001,
'reso': 1.7100000000000002,
'type': 'HpBoxcar',
'order': 1,
'frequency': 30.777777777777786,
'saturate': false
});
rep.set({
'length': 1,
'points': [
[
-113,
0.8166666666666667
],
[
4492,
-2.033333333333333
],
[
5465,
0.5999999999999999
],
[
13664,
1.1
]
],
'monophonic': false,
'gain': 1
});
del.set({
'feedback': 0.29,
'time': 0.15511,
'dry': 1,
'wet': 0.5
});
osc5.set({
'amplitude': 5.551115123125783e-17,
'bias': 0,
'length': 1,
'frequency': 2,
'phase': 0,
'shape': 'noise'
});
vol.set({
'preamp': 6.189999999999996,
'center': 16.519999999999982
});
osc3.set({
'amplitude': 0.58,
'bias': 0,
'length': 1,
'frequency': 39.111111111111114,
'phase': 0,
'shape': 'sin'
});
mix.set({
'amplitude': 1,
'levela': 0.25,
'levelb': 0.25,
'levelc': 0.25,
'leveld': 0.25,
'normalize': false,
'saturate': true
});
osc.getInterface().autoZoom();
fil.getInterface().autoZoom();
rep.getInterface().autoZoom();
del.getInterface().autoZoom();
osc5.getInterface().autoZoom();
vol.getInterface().autoZoom();
osc3.getInterface().autoZoom();
mix.getInterface().autoZoom();